Results of the wxWidgets 2.8.10 UNICODE build
16-Sep-2009 by Hirofumi Fujii
These pictures are the results of the "Hello world in Japanese" (source code is show blow) by
using wxWidgets 2.8.10 UNICODE build. The top row is the initial images, the second one
is the menu selections, and the third one is the dialog windows.
Windows MSYS/MinGW | Linux GTK1 | Linux GTK2
|
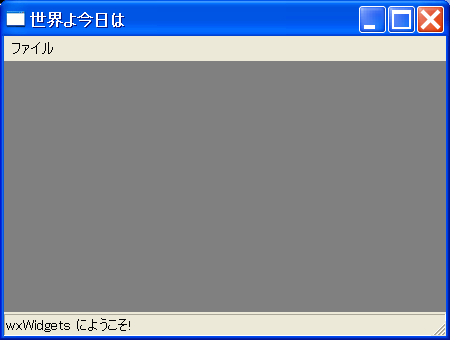 | 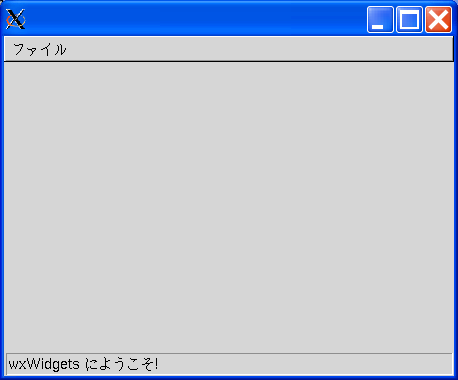 |
|
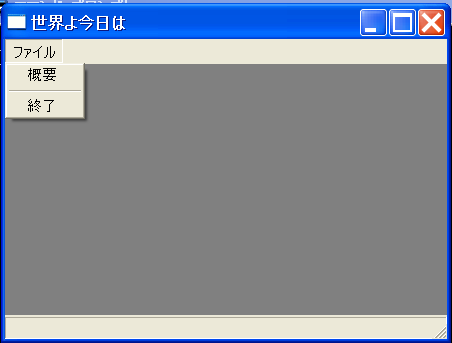 | 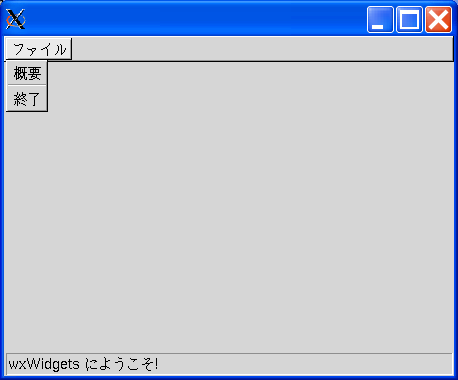 |
|
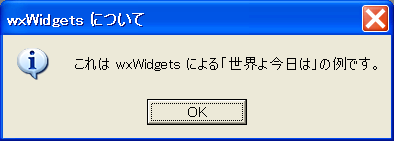 | 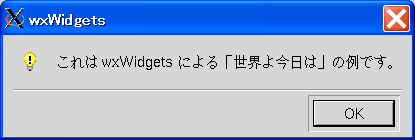 |
|
Source code
This is the source code of the "Hello world in Japanese" based on
the
Robert Roebling's "Hello world".
// jworld.cpp
#include <wx/wx.h>
#include <string>
class MyApp: public wxApp
{
virtual bool OnInit();
};
class MyFrame: public wxFrame
{
public:
MyFrame(const wxString& title, const wxPoint& pos,
const wxSize& size);
void OnQuit(wxCommandEvent& event);
void OnAbout(wxCommandEvent& event);
DECLARE_EVENT_TABLE()
};
class MyText
{
public:
static const wchar_t m_frame_title[];
static const wchar_t m_menu_file[];
static const wchar_t m_menu_about[];
static const wchar_t m_menu_exit[];
static const wchar_t m_status[];
static const wchar_t m_about_title[];
static const wchar_t m_about[];
};
enum
{
ID_Quit = 1,
ID_About,
};
BEGIN_EVENT_TABLE(MyFrame, wxFrame)
EVT_MENU(ID_Quit, MyFrame::OnQuit)
EVT_MENU(ID_About, MyFrame::OnAbout)
END_EVENT_TABLE()
IMPLEMENT_APP(MyApp)
const wchar_t MyText::m_frame_title[] =
{0x4e16,0x754c,0x3088,0x4eca,0x65e5,0x306f,0x0000};
const wchar_t MyText::m_menu_file[] =
{0x30d5,0x30a1,0x30a4,0x30eb,0x0000};
const wchar_t MyText::m_menu_about[] = {0x6982,0x8981,0x0000};
const wchar_t MyText::m_menu_exit[] = {0x7d42,0x4e86,0x0000};
const wchar_t MyText::m_status[] =
{0x0077,0x0078,0x0057,0x0069,0x0064,0x0067,0x0065,0x0074,0x0073,
0x0020,
0x306b,0x3088,0x3046,0x3053,0x305d,0x0021,0x0000};
const wchar_t MyText::m_about_title[] =
{0x0077,0x0078,0x0057,0x0069,0x0064,0x0067,0x0065,0x0074,0x0073,
0x0020,
0x306b,0x3064,0x3044,0x3066,0x0000};
const wchar_t MyText::m_about[] =
{0x3053,0x308c,0x306f,0x0020,
0x0077,0x0078,0x0057,0x0069,0x0064,0x0067,0x0065,0x0074,0x0073,
0x0020,
0x306b,0x3088,0x308b,0x300c,
0x4e16,0x754c,0x3088,0x4eca,0x65e5,0x306f,
0x300d,0x306e,0x4f8b,0x3067,0x3059,0x3002,0x0000};
bool
MyApp::OnInit()
{
MyFrame* frame = new MyFrame(
MyText::m_frame_title, wxPoint(50,50),
wxSize(450,340));
frame->Show(TRUE);
SetTopWindow(frame);
return true;
}
MyFrame::MyFrame(const wxString& title,
const wxPoint& pos, const wxSize& size) :
wxFrame((wxFrame*)0, -1, title, pos, size)
{
wxMenu* menuFile = new wxMenu;
menuFile->Append(ID_About, MyText::m_menu_about);
menuFile->AppendSeparator();
menuFile->Append(ID_Quit, MyText::m_menu_exit);
wxMenuBar* menuBar = new wxMenuBar;
menuBar->Append(menuFile, MyText::m_menu_file);
SetMenuBar(menuBar);
CreateStatusBar();
SetStatusText(MyText::m_status);
}
void
MyFrame::OnQuit(wxCommandEvent& WXUNUSED(event))
{
Close(true);
}
void
MyFrame::OnAbout(wxCommandEvent& WXUNUSED(event))
{
wxMessageBox(MyText::m_about, MyText::m_about_title,
wxOK | wxICON_INFORMATION, this);
}